Character
Functions
Built-in
Library Functions for Character Classifications and Conversion
|
Required header: #include
<ctype.h>
C++ uses the American Standard Code for Information
Interchange (ASCII) character set. The CTYPE library includes character
classification functions. A character
is passed to the functions and the functions return
values that can be stored or printed.
Basic ASCII Categories:
Category |
ASCII Characters |
Uppercase letters |
'A' through 'Z' |
Lowercase letters |
'a' through 'z' |
Digits (0 through 9) |
'0' through '9' |
Whitespace |
Space, tab, line feed(newline), and carriage
return |
Punctuation |
!"#$%&'()*+,-./:;<=>?@[\]^_{|}~ |
Blank space |
The blank space character |
Most common CTYPE functions:
1. isalnum( ) |
returns a TRUE (nonzero) if the argument is digit 0-9,
or an alphabetic character (alphnumeric).
Otherwise returns FALSE. |
2. isalpha( ) |
returns TRUE if the argument is an upper or lower case
letter. |
3. isascii( ) |
returns TRUE if the integer argument is in the ASCII
range 0-127. Treats 128-255 as non-ASCII. |
You should use isascii( ) to verify
that an integer value is indeed a valid ASCII character before using
any of the functions #4 through #9. |
4. isdigit( ) |
returns TRUE if the argument
is a digit 0 - 9 |
5. isgraph( ) |
returns TRUE if the argument
is any printable character from ASCII 32 to 127, except the space. |
6. islower( ) |
returns TRUE if the argument
is a lowercase letter. |
7. isupper( ) |
returns TRUE if the argument
is an uppercase leter. |
8. ispunct( ) |
returns TRUE if the argument
is any punctuation character (see chart above). |
9. isspace( ) |
returns TRUE if the argument
is a whitespace (see chart above). |
The following character functions are
conversion functions.
toascii( ) |
converts the argument (an arbitrary integer) to a valid
ASCII character number 0-127.
c =
toascii(500); //c gets number 116
// (modulus 500%128)
c = toascii('d'); //c gets number 100 |
tolower( ) |
converts the argument (an uppercase ASCII character) to
lowercase.
c = tolower('Q'); // c becomes 'q' |
toupper( ) |
converts the argument (a lowercase ASCII character) to
uppercase.
c = toupper('q'); //c becomes 'Q' |
**Note:
tolower( ) and toupper( ) will actually check to see if the argument
is the appropriate uppercase or lowercase before making the
conversion. |
|
|
Warning:
You cannot pass an entire string to character
functions. If you want to test the elements of a string, you
must pass the string one element at a time. |
Note:
Even though these functions' prototypes specify an integer argument, you
may pass a single character variable when you
call it. If you pass an integer to these functions,
the functions will act upon the corresponding ASCII character associated
with your integer.
(i.e. int i = 65; and
char j = 'A'; are the same.)
|
The first 128 ASCII
characters (0-127) are universal. Character codes 128-255 may not
be available -- check your system! |
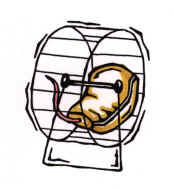
|