Return to Topic Menu
|
Computer Science Main Page |
MathBits.com
|
Terms of Use
|
Resource CD
Demo Program -
Arrays and Functions |
Task: This assignment will be an "upgrade" of the previous demo with
the use of functions. Create a function to fill an array with 25 grades.
Create a function to
print the array. Create a function to find the average of the grades and
return the average to main.
|
#include <iostream.h>
#include <stdlib.h>
#include <apvector.h>
void fill_it(apvector <int>&grades);
void print_it(apvector <int>&grades);
double average(apvector <int>&grades);
int main(void)
{
system ("CLS");
apvector <int> grades(25);
//Call function to Fill array
fill_it(grades);
//Call function to Print the
array
print_it(grades);
//Call function to average the
grades
double avg = average(grades);
cout<<"The average of the grades: " << avg;
cout<<endl<<endl;
return 0;
}
//Function to fill array
void fill_it(apvector <int>&grades)
{
int size = grades.length();
for (int i = 0; i < size; i++)
{
cout<<"Please enter
grade: ";
cin>> grades[i];
}
return;
}
//Function to print array
void print_it(apvector <int>&grades)
{
int len = grades.length( );
for(int i = 0; i < len; i++)
{
cout<<grades[i] << endl;
}
return;
}
//Function to average the grades
double average(apvector <int>&grades)
{
int total = 0;
double avg;
int len = grades.length( );
for(int i = 0; i < len; i++)
{
total += grades[i];
}
avg = (double) total/len; //avoid
integer division
return avg;
}
|
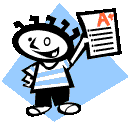
|
|